How To Replace Text In Multiple Files Using A Bash Script In Linux
- Chris
- Mar 31
- 2 min read
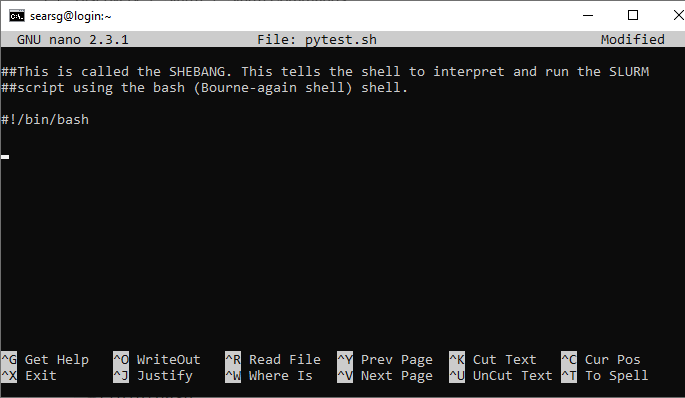
If you have many files with outdated information, updating them manually is time-consuming.
Whether it's fixing outdated file paths, renaming variables, or modifying config values, Bash provides powerful tools like grep, sed, and find to automate this task.
In this post, I will show you how to replace text across multiple files efficiently using a Bash script.
Why and When Use Bash for Bulk Text Replacement?
Automating text replacement is useful for:
Updating variable names in code files
Changing file paths in scripts.
Fixing incorrect configuration settings.
Renaming project names in documentation.
Instead of editing each file manually, a Bash script can do it in seconds.
Allow me to explain it with an example Bash script.
Problem: Bulk Replace Paths in Multiple Files
Problem: Bulk Replace Paths in Multiple Files
Imagine you have multiple files referring to old log file paths:
Old Path: ~/data/logs/app.log
New Path: ~/data/log/app.log
Instead of searching and replacing each instance manually, let’s automate it.
Step 1: Create a Test Directory and Sample Files
First, create a test environment before modifying real files.
Create a directory in your home folder:
mkdir -p ~/replace_test && cd ~/replace_test
Create sample files containing the old path:
echo 'Log file: ~/data/logs/app.log' > script1.sh
echo 'ERROR_LOG="~/data/logs/app.log"' > config.env
echo 'echo "Processing ~/data/logs/app.log"' > process.sh
Verify occurrences of the old path:
grep "~/data/logs/app.log" *
Sample Output:
config.env:ERROR_LOG="~/data/logs/app.log"
process.sh:echo "Processing ~/data/logs/app.log"
script1.sh:Log file: ~/data/logs/app.log
Step 2: Replace Text in Multiple Files Using a Bash Script
Now, create a script named replace_text.sh:
#!/usr/bin/env bash
if [[ $# -ne 3 ]]; then
echo "Usage: $0 <old_path> <new_path> <directory>"
exit 1
fi
OLD_PATH=$(printf '%s\n' "$1" | sed 's/[\/&]/\\&/g')
NEW_PATH=$(printf '%s\n' "$2" | sed 's/[\/&]/\\&/g')
SEARCH_DIR=$3
echo "Replacing occurrences of: $1 -> $2 in $SEARCH_DIR"
# Find and replace text safely
find "$SEARCH_DIR" -type f -exec sed -i "s/$OLD_PATH/$NEW_PATH/g" {} +
echo "Replacement completed."
Step 3: Make the Script Executable
chmod +x replace_text.sh
Step 4: Run the Script
Execute the script to replace all occurrences of ~/data/logs/app.log with ~/data/log/app.log:
./replace_text.sh "~/data/logs/app.log" "~/data/log/app.log" ~/replace_test
Sample output:
Replacing occurrences of: ~/data/logs/app.log -> ~/data/log/app.log in /home/ostechnix/replace_test
Replacement completed.
Step 5: Verify the Changes
Run:
grep "~/data/log/app.log" *
Sample Output:
config.env:ERROR_LOG="~/data/log/app.log"
process.sh:echo "Processing ~/data/log/app.log"
script1.sh:Log file: ~/data/log/app.log
Ensure no old path remains:
grep "~/data/logs/app.log" *
If there's no output, the replacement worked.
This script:
Handles Special Characters: Escapes /, &, and other symbols correctly.
Uses find -exec sed: Safer than grep | xargs sed.
Efficient for Large Projects: Works on many files without excessive CPU usage.
You can now use this script on real files.
Precautions Before Running it on Real Files:
Backup Your Files: Always create a backup before making bulk changes
Test on a Small Sample: Run it on a test directory first, as we did with
~/replace_test
Dry Run with grep: Check how many files will be affected.
To do it, you can run
grep -rl "~/data/logs/app.log" ~/replace_test/
Manually Inspect Changes: After running the script, verify a few modified files.
Conclusion
In this tutorial, we learned how to replace text in multiple files using a simple Bash script.
We have explained how this script automates path updates in multiple files, preventing errors and saving time with a practical example. Always test with backups before running on critical files.
Comments